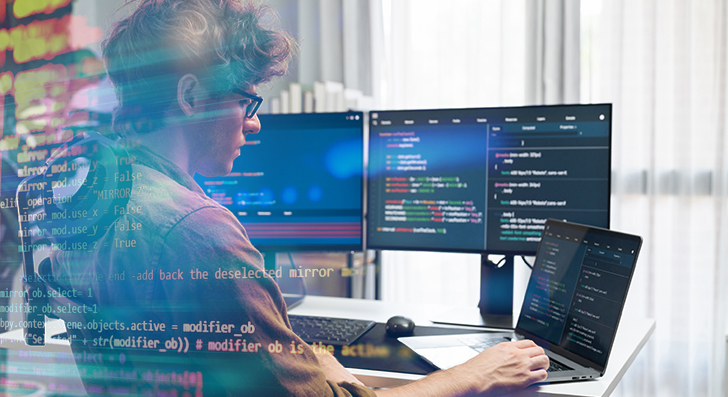
Scalability implies your software can handle advancement—additional end users, a lot more data, and more targeted visitors—devoid of breaking. Like a developer, developing with scalability in your mind saves time and worry later on. Here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability is not a little something you bolt on later—it ought to be element within your program from the start. Several purposes fall short every time they increase fast due to the fact the original layout can’t manage the additional load. To be a developer, you'll want to Feel early regarding how your process will behave under pressure.
Get started by coming up with your architecture for being adaptable. Prevent monolithic codebases the place almost everything is tightly linked. As an alternative, use modular style or microservices. These styles break your application into smaller, impartial parts. Each module or company can scale on its own devoid of influencing the whole system.
Also, think of your databases from working day one. Will it require to manage one million buyers or merely 100? Pick the correct sort—relational or NoSQL—determined by how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t want them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than current conditions. Consider what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that assistance scaling, like message queues or function-driven techniques. These aid your app deal with much more requests with out getting overloaded.
When you build with scalability in mind, you're not just making ready for fulfillment—you happen to be minimizing foreseeable future head aches. A nicely-prepared procedure is simpler to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct databases can be a vital Component of constructing scalable programs. Not all databases are built a similar, and using the Incorrect you can sluggish you down or even induce failures as your application grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are definitely sturdy with relationships, transactions, and regularity. They also aid scaling tactics like study replicas, indexing, and partitioning to take care of far more visitors and data.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing substantial volumes of unstructured or semi-structured information and might scale horizontally extra very easily.
Also, take into consideration your study and produce patterns. Have you been accomplishing plenty of reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may take care of significant write throughput, and even event-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need Superior scaling characteristics now, but choosing a database that supports them indicates you gained’t want to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details based on your accessibility designs. And generally observe databases general performance when you mature.
To put it briefly, the right databases depends on your application’s composition, speed needs, and how you expect it to grow. Take time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Rapid code is vital to scalability. As your app grows, each little hold off provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you build economical logic from the beginning.
Commence by creating clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most intricate Remedy if an easy 1 is effective. Maintain your functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes as well extensive to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These often sluggish things down in excess of the code itself. Be sure Every single query only asks for the information you truly want. Stay clear of Pick *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing a lot of joins, especially across substantial tables.
In the event you observe the Gustavo Woltmann news same info remaining requested over and over, use caching. Retail outlet the outcomes briefly applying instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations once you can. In lieu of updating a row one after the other, update them in groups. This cuts down on overhead and helps make your app additional effective.
Remember to take a look at with substantial datasets. Code and queries that work good with one hundred information may possibly crash every time they have to take care of 1 million.
In short, scalable apps are quick apps. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application stay easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers plus more traffic. If everything goes through 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments enable keep the application rapidly, secure, and scalable.
Load balancing spreads incoming website traffic across several servers. As opposed to 1 server doing all the do the job, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When end users request the same facts once more—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and will make your app extra productive.
Use caching for things that don’t transform frequently. And constantly make sure your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your application manage additional users, continue to be quick, and Recuperate from complications. If you plan to expand, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you'll need equipment that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t should invest in components or guess future capacity. When visitors raises, you may insert additional means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and stability instruments. You may center on making your application in place of taking care of infrastructure.
Containers are One more essential Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, from your notebook on the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into solutions. You could update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, start off applying these equipment early. They save time, minimize hazard, and enable you to keep centered on developing, not repairing.
Observe Every thing
When you don’t monitor your application, you gained’t know when things go Improper. Checking can help the thing is how your app is undertaking, spot problems early, and make greater conclusions as your application grows. It’s a key Portion of making scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app way too. Control just how long it will require for people to load web pages, how frequently glitches transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you must get notified quickly. This aids you repair problems fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh feature and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it triggers genuine destruction.
As your application grows, visitors and info increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be in control.
In short, checking helps you maintain your app trusted and scalable. It’s not just about recognizing failures—it’s about knowing your system and making certain it really works effectively, even stressed.
Last Views
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking stressed. Commence smaller, think huge, and Establish intelligent.